Developing AI Agents with NodeJS: A Practical Guide
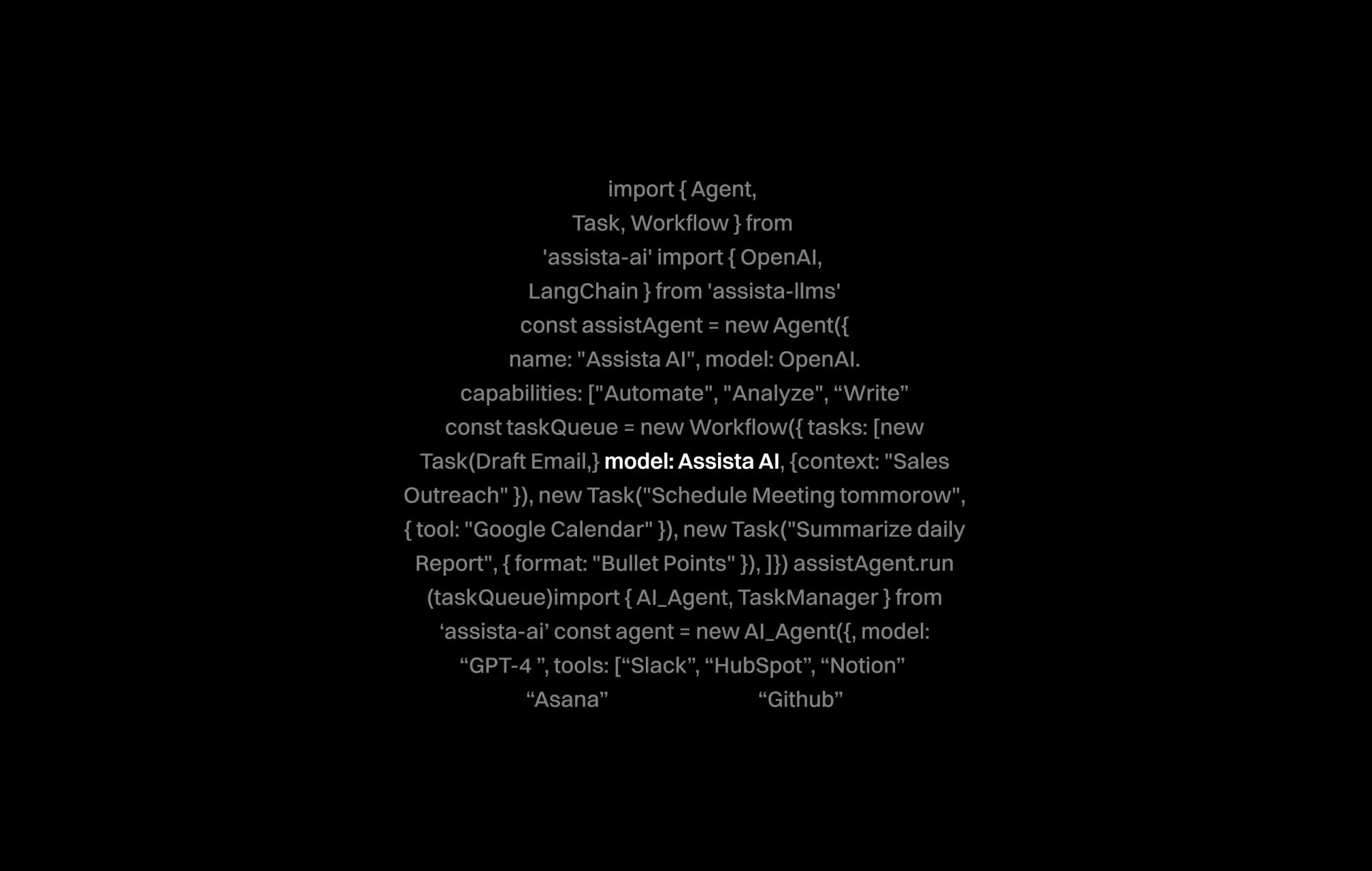
Jumping into AI Agent NodeJS Development
If you’re looking to build an AI agent nodejs, you might feel overwhelmed by the myriad of options and technical considerations. You may be grappling with questions like: How do I set up my environment? Which libraries should I use? And most importantly, how can I deploy a robust solution quickly? This guide is designed for developers who want to harness the power of NodeJS to create practical and reliable AI agents. Over the next sections, you will discover why NodeJS is an excellent choice, how to set up your development environment, and step-by-step instructions on building your first AI agent. You will also learn essential best practices, tips, and integration strategies that make your solution flexible and scalable. Whether you are a seasoned developer or just starting out, this guide offers insights and actionable examples tailored for your success.
Why Choose NodeJS for AI Agent Development?
NodeJS has emerged as a powerful platform for AI agent nodejs development because it combines fast performance with a rich ecosystem of packages. Using NodeJS allows you to build lightweight yet effective AI solutions that respond to real-time data and user inputs. Many developers appreciate its non-blocking, event-driven model, which is ideal for managing multiple tasks concurrently—an essential feature when dealing with AI agents that integrate various data streams.
Key Advantages of NodeJS
- Performance: NodeJS’s event-driven architecture handles multiple operations concurrently, crucial for processing user requests and data analysis.
- Vibrant Ecosystem: With npm, you gain access to thousands of packages that simplify many AI development tasks.
- Ease of Use: JavaScript’s familiarity for many developers makes it easier to build, test, and deploy AI agents rapidly.
Using NodeJS minimizes development friction and speeds up the process of converting conceptual AI workflows into working prototypes. By leveraging community libraries and resources like those available on the integrations page, you can further expand the potential of your project. In the next section, we explore how to set up your environment so that you’re ready to code your first AI agent.
Setting Up Your NodeJS Development Environment
Before you dive into coding your AI agent nodejs, it’s important to have your development environment properly configured. Setting up NodeJS, installing necessary libraries, and preparing your workspace are the foundational steps to ensure a smooth development journey.
Essential Tools and Software
You will need a few critical tools to get started:
- NodeJS: Download and install the latest stable release from the official website.
- Code Editor: Use Visual Studio Code, Atom, or any editor that supports JavaScript development.
- Version Control: Git is essential for managing your code changes and collaborating with others.
Environment Setup Steps
Follow these steps to get your NodeJS environment ready:
- Install NodeJS from the official site.
- Initialize your project using
npm init
. - Install necessary libraries, such as
express
for web server capabilities oraxios
for making API requests. - Create a basic file structure that separates code, configuration, and assets.
This structured approach not only prepares you for development but also fosters a more organized codebase. For more details on managing your projects and staying updated on improvements, you might want to check out the changelog on Assista’s website. With your environment ready, you can now focus on building your first AI agent.
Building Your First AI Agent with NodeJS
Now it’s time to put your environment to work by creating an AI agent nodejs. In this section, you will find a step-by-step guide complete with code examples that illustrate how to develop a simple AI agent. This hands-on approach means you get to experience the process from coding to execution, ensuring you have a working prototype by the end.
Step-by-Step Walkthrough
Below is a practical example to help you get started:
- Initialize Your Project:
Open your terminal and run:npm init -y
- Install Required Libraries:
For instance, install Express and any other library required for your agent:npm install express axios
- Create Your Main File:
Create aserver.js
file and set up a basic Express server to handle AI requests. - Build the AI Agent Logic:
In your code, create functions that process user input, call external APIs if needed, and return a response. Here is a simplified example:// server.js const express = require('express'); const axios = require('axios'); const app = express(); app.use(express.json()); // Sample endpoint for your AI agent app.post('/agent', async (req, res) => { const userInput = req.body.input; // Process the input here, for instance making an API call try { // Example external API call - replace with your logic const result = await axios.get('https://api.example.com/process', { params: { query: userInput } }); res.json({ result: result.data }); } catch (error) { res.status(500).json({ error: 'Internal Server Error' }); } }); app.listen(3000, () => { console.log('AI agent nodejs server is running on port 3000'); });
- Test Your Agent:
Use tools like Postman to simulate requests and validate that your agent processes the input as expected.
This example is intentionally simple to provide a foundation. As you expand your agent, you can integrate more advanced features like natural language processing libraries or specialized machine learning models. Refer to the Assista homepage for more insights on how innovative solutions in this space are built. The following section will dive into best practices to help you refine your project further.
Best Practices and Tips for Developing Robust AI Agents
Creating a basic AI agent is simply the beginning; making it robust and production-ready requires care and attention. This section covers best practices and practical tips to ensure your AI agent nodejs stands out in terms of performance, reliability, and security.
Development Best Practices
To build a resilient AI agent, consider the following strategies:
- Modularity: Break your code into functions and modules so that each part handles a specific responsibility. This approach simplifies future maintenance and updates.
- Error Handling: Ensure that you catch and handle errors gracefully. Logging errors and providing clear feedback will facilitate debugging.
- Security: Validate user input and secure your API endpoints. Since AI agents often interact with external systems, safeguarding against vulnerabilities is essential.
- Documentation: Maintain clear documentation for your functions and modules to help team members understand the codebase.
Practical Tips for AI Agent NodeJS
Below are some practical tips that developers have found useful:
- Organize your code into layers such as input handling, processing, and output response.
- Use environment variables to manage configuration settings like API keys and endpoints.
- Implement logging and performance monitoring to track metrics and optimize performance.
- Regularly review and update dependencies to use the latest, secure versions.
These practices help you build an AI agent nodejs that not only performs well under load but is also easier to scale and maintain. For further technical insights and advanced updates, check out the about page to learn more about the team behind these innovations. With your coding practices in place, the next step is to integrate your agent with various external platforms.
Integrating Your AI Agent with Multiple Platforms
Integrating your AI agent nodejs with other platforms is the key to unlocking its full potential. Today’s business environments demand connectivity across various tools and services, and a well-integrated agent can perform complex tasks across multiple systems efficiently.
Why Integration Matters
Integrations allow your AI agent to:
- Fetch and process real-time data from external sources.
- Execute actions across productivity tools such as Gmail, Trello, and Google Sheets.
- Provide seamless notifications and reports on business operations.
For example, an effective AI agent might retrieve leads from HubSpot, update tasks in Asana, and even post updates on Slack. By connecting with services that you already use, you can significantly boost your team’s productivity and accuracy in decision-making. Assista is a great example of a platform that empowers non-technical users to automate such multi-step workflows. You can see a real-world implementation by exploring the marketplace, where prebuilt automations are available to extend functionality.
Integration Best Practices
When planning integrations, keep the following in mind:
- Authentication: Use OAuth to securely connect your agent with external services. Many platforms such as Gmail and Salesforce rely on OAuth for safe data access.
- Modular API Calls: Write separate modules to handle API requests for each service. This improves code clarity and makes troubleshooting easier.
- Data Consistency: Ensure that the data you fetch from one platform is transformed properly before being sent to another. Consistency is key in maintaining data integrity across systems.
By following these guidelines, you can create a robust network of integrations that enhances the overall utility of your AI agent. This approach not only builds flexibility into your code but also equips your solution to adapt as new tools emerge in the market. If you are eager to see innovative automation in action, consider checking out how Assista connects with top productivity tools, a feature that has helped many businesses boost their operational efficiency.
Wrapping Up Your AI Agent Journey
In this guide, you learned how to develop an AI agent nodejs from setting up your development environment to building a functional prototype and integrating it with multiple platforms. We explored why NodeJS is ideal for rapid agent development, walked through setting up critical tools, and provided step-by-step instructions with real code examples. We also shared best practices regarding modularity, error handling, and security. Finally, the importance of integrating your AI agent with popular services such as Gmail, Trello, and Google Sheets was highlighted to help you build a versatile solution.
Your journey in building powerful AI agents doesn’t have to stop here. With the right tools and approaches, you can expand on this foundation to create even more sophisticated workflow automations. If you’re ready to take your project to the next level, consider signing up for an account with Assista, where you can explore additional resources, prebuilt automations, and community support. Embrace the opportunities and start transforming your digital operations today!
Join over 1,400 professionals who reclaimed their time and sanity by letting AI handle the busywork.